Calculate the Power of a Number in Python
In Python, calculating the power of a number is a common mathematical operation. Whether you’re working with exponents in mathematical functions or performing scientific calculations, raising a number to a power is essential. Python provides several ways to calculate the power of a number, from basic operators to built-in functions.
In this blog, we’ll explore different methods to compute the power of a number using Python, from simple calculations to more advanced techniques.
What Does Power Mean in Mathematics?
When we say “power,” we refer to raising a number (called the base) to the exponent (or power). For example:
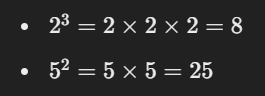
Here, the base is the number that is being multiplied, and the exponent indicates how many times the base is multiplied by itself.
Method 1: Using the Exponentiation Operator (**
)
Python provides an exponentiation operator (**
) that makes it simple to calculate the power of a number. This operator raises the base to the power of the exponent.
Code Example:
# Program to calculate power using the exponentiation operator
# Step 1: Define the base and exponent
base = 2
exponent = 3
# Step 2: Use the exponentiation operator (**) to calculate power
result = base ** exponent
# Step 3: Print the result
print(f"{base} raised to the power of {exponent} is: {result}")
Explanation:
- Base and Exponent: We define the
base
and theexponent
values that we want to use. - Exponentiation Operator: The operator
**
raises the base to the power of the exponent. - Output: The result is printed to the console.
Output:
2 raised to the power of 3 is: 8
Method 2: Using the pow()
Function
Python also has a built-in pow()
function that can be used to calculate powers. It takes two arguments: the base and the exponent, and returns the result.
Code Example:
# Program to calculate power using the pow() function
# Step 1: Define the base and exponent
base = 5
exponent = 2
# Step 2: Use the pow() function to calculate power
result = pow(base, exponent)
# Step 3: Print the result
print(f"{base} raised to the power of {exponent} is: {result}")
Explanation:
- pow() Function: The
pow()
function takes two arguments: the base and the exponent, and returns the base raised to the power of the exponent. - Output: The result is printed, showing the power calculation.
Output:
5 raised to the power of 2 is: 25
Method 3: Using a Custom Function
If you want more control over the power calculation or need to use this operation in multiple places, you can define a custom function to calculate the power of a number.
Code Example:
# Program to calculate power using a custom function
# Step 1: Define a function to calculate power
def calculate_power(base, exponent):
result = 1
for _ in range(exponent):
result *= base
return result
# Step 2: Define the base and exponent
base = 3
exponent = 4
# Step 3: Call the function and print the result
result = calculate_power(base, exponent)
print(f"{base} raised to the power of {exponent} is: {result}")
Explanation:
- Function Definition: The function
calculate_power()
takesbase
andexponent
as arguments and calculates the power using a loop. - Loop: The loop multiplies the base by itself,
exponent
number of times. - Result: The result is returned and printed to the console.
Output:
3 raised to the power of 4 is: 81
Method 4: Using the math.pow()
Function
Python’s math
module provides another way to calculate the power of a number with the math.pow()
function. This function works similarly to the pow()
function, but it always returns a float value.
Code Example:
# Program to calculate power using the math.pow() function
# Step 1: Import the math module
import math
# Step 2: Define the base and exponent
base = 2
exponent = 5
# Step 3: Use the math.pow() function to calculate power
result = math.pow(base, exponent)
# Step 4: Print the result
print(f"{base} raised to the power of {exponent} is: {result}")
Explanation:
- math.pow(): The
math.pow()
function is part of themath
module and returns the result as a float. - Float Result: Even though the calculation is integer-based, the result is returned as a floating-point number.
- Output: The result is printed to the console.
Output:
2 raised to the power of 5 is: 32.0
Method 5: Using Recursion
If you want to explore a more advanced technique, you can calculate the power of a number using recursion. Recursion is a process where a function calls itself to break down a problem into smaller sub-problems.
Code Example:
# Program to calculate power using recursion
# Step 1: Define a recursive function to calculate power
def power(base, exponent):
if exponent == 0:
return 1
else:
return base * power(base, exponent - 1)
# Step 2: Define the base and exponent
base = 4
exponent = 3
# Step 3: Call the function and print the result
result = power(base, exponent)
print(f"{base} raised to the power of {exponent} is: {result}")
Explanation:
- Base Case: The recursion ends when the exponent reaches zero, returning 1 (since any number raised to the power of 0 is 1).
- Recursive Call: The function calls itself, reducing the exponent by 1 each time until the base case is reached.
- Output: The result is calculated and printed.
Output:
4 raised to the power of 3 is: 64
Conclusion
In this blog, we explored various ways to calculate the power of a number in Python:
- Using the exponentiation operator (
**
) for quick and simple power calculations. - Utilizing the
pow()
function to calculate power in a more function-driven way. - Writing a custom function for flexibility and reusability.
- Leveraging Python’s
math.pow()
function for advanced calculations. - Applying recursion for a more complex and theoretical approach.
Each method has its own use cases, depending on the situation or preference. Practice these methods to deepen your understanding of power calculations in Python!
Stay tuned for more Python tutorials! 😊