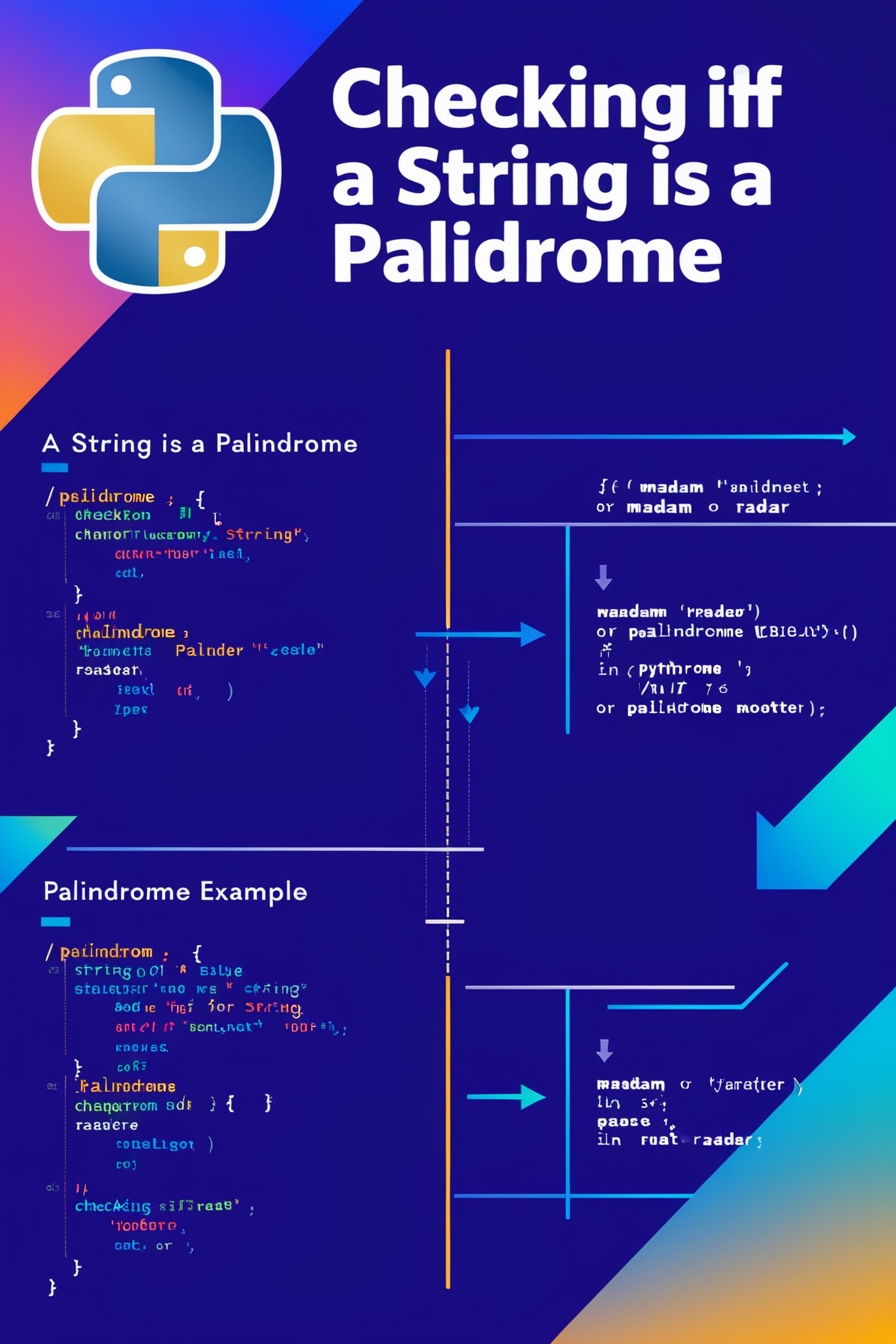
Check if a String is a Palindrome in Python
A palindrome is a word, phrase, number, or sequence of characters that reads the same backward as forward. Checking if a string is a palindrome is a common coding problem, and Python offers several simple ways to solve it.
In this blog, we’ll explore different methods to check if a string is a palindrome in Python, including using slicing, loops, and recursion.
What is a Palindrome?
In the context of strings, a palindrome is a sequence of characters that reads the same backward as forward. Some examples of palindrome strings are:
- “madam” → Reversed: “madam” (Palindrome ✅)
- “racecar” → Reversed: “racecar” (Palindrome ✅)
- “python” → Reversed: “nohtyp” (Not a Palindrome ❌)
Method 1: Using String Slicing
Python’s string slicing feature provides an easy way to check if a string is a palindrome by reversing the string and comparing it with the original string.
Code Example:
# Program to check if a string is a palindrome using slicing
# Step 1: Define a function to check palindrome
def is_palindrome(s):
# Reverse the string using slicing
reversed_s = s[::-1]
# Step 2: Check if the original string is equal to the reversed string
return s == reversed_s
# Step 3: Test the function
string = "madam"
if is_palindrome(string):
print(f"'{string}' is a palindrome")
else:
print(f"'{string}' is not a palindrome")
Explanation:
- String Slicing: We reverse the string using Python’s slicing feature
[::-1]
. - Comparison: The original string is compared with its reversed version. If they are equal, the string is a palindrome.
- Output: The result is printed based on the comparison.
Output:
'madam' is a palindrome
Method 2: Using a Loop
If you prefer a manual approach, you can use a loop to check if a string is a palindrome by comparing characters from the beginning and end of the string one by one.
Code Example:
# Program to check if a string is a palindrome using a loop
# Step 1: Define a function to check palindrome using a loop
def is_palindrome(s):
# Step 2: Use two pointers to compare characters from the start and end
start = 0
end = len(s) - 1
while start < end:
if s[start] != s[end]:
return False
start += 1
end -= 1
return True
# Step 3: Test the function
string = "racecar"
if is_palindrome(string):
print(f"'{string}' is a palindrome")
else:
print(f"'{string}' is not a palindrome")
Explanation:
- Two Pointers: We use two pointers,
start
andend
, to compare the characters from the beginning and the end of the string. - Character Comparison: If the characters at
start
andend
don’t match, the string is not a palindrome. - Output: The result is printed based on the comparison.
Output:
'racecar' is a palindrome
Method 3: Using Recursion
Recursion can be used to check if a string is a palindrome by comparing the first and last characters of the string, and then recursively checking the substring in between.
Code Example:
# Program to check if a string is a palindrome using recursion
# Step 1: Define a recursive function to check palindrome
def is_palindrome_recursive(s):
# Base case: if the string is empty or has one character, it is a palindrome
if len(s) <= 1:
return True
# Step 2: Compare the first and last characters
if s[0] == s[-1]:
# Recursively check the substring without the first and last characters
return is_palindrome_recursive(s[1:-1])
else:
return False
# Step 3: Test the function
string = "level"
if is_palindrome_recursive(string):
print(f"'{string}' is a palindrome")
else:
print(f"'{string}' is not a palindrome")
Explanation:
- Base Case: If the string is empty or has only one character, it is a palindrome.
- Recursive Call: We compare the first and last characters. If they match, we recursively check the substring formed by removing those characters.
- Output: The result is printed based on the recursive comparison.
Output:
'level' is a palindrome
Method 4: Ignoring Case and Special Characters
Often, when checking for palindromes, we want to ignore case sensitivity and special characters such as spaces, punctuation, etc. In this case, we can clean the string by removing non-alphanumeric characters and converting everything to lowercase.
Code Example:
# Program to check if a string is a palindrome while ignoring case and special characters
import re
# Step 1: Define a function to clean and check for palindrome
def is_palindrome(s):
# Clean the string: remove non-alphanumeric characters and convert to lowercase
cleaned_string = re.sub(r'[^a-zA-Z0-9]', '', s).lower()
# Step 2: Check if the cleaned string is equal to its reversed version
return cleaned_string == cleaned_string[::-1]
# Step 3: Test the function
string = "A man, a plan, a canal: Panama"
if is_palindrome(string):
print(f"'{string}' is a palindrome")
else:
print(f"'{string}' is not a palindrome")
Explanation:
- Cleaning the String: We use the
re.sub()
function to remove all non-alphanumeric characters and convert the string to lowercase. - String Slicing: We compare the cleaned string with its reversed version.
- Output: The result is printed based on the comparison.
Output:
'A man, a plan, a canal: Panama' is a palindrome
Method 5: Using the reversed()
Function
Python’s built-in reversed()
function can also be used to check for palindromes. It allows you to reverse any sequence, including strings, without needing slicing.
Code Example:
# Program to check if a string is a palindrome using the reversed() function
# Step 1: Define a function to check palindrome using reversed()
def is_palindrome(s):
# Compare the string with its reversed version using the reversed() function
return list(s) == list(reversed(s))
# Step 2: Test the function
string = "deified"
if is_palindrome(string):
print(f"'{string}' is a palindrome")
else:
print(f"'{string}' is not a palindrome")
Explanation:
- Reversing the String: We use
reversed()
to reverse the string. - Comparison: We compare the original string with the reversed version.
- Output: The result is printed based on the comparison.
Output:
'deified' is a palindrome
Conclusion
In this blog, we explored various methods to check if a string is a palindrome in Python:
- String Slicing: A simple and concise way to reverse a string and compare it.
- Loop: A manual approach using two pointers.
- Recursion: A recursive technique to check for palindromes.
- Ignoring Case and Special Characters: A method that handles case sensitivity and special characters.
- Using
reversed()
: Python’s built-in function to reverse sequences.
Each method has its own use cases and trade-offs. Depending on your requirements, you can use any of these techniques to check for palindrome strings in Python. Happy coding! 😊
Stay tuned for more Python tutorials!