Find the Sum of N Natural Numbers in Python
Finding the sum of the first N
natural numbers is a common and simple programming problem that helps beginners learn how to work with loops, formulas, and arithmetic operations in Python. In this blog, we’ll cover multiple ways to calculate the sum of the first N
natural numbers.
By the end of this post, you’ll know:
- What natural numbers are.
- How to calculate the sum of natural numbers using Python.
- Different methods to solve this problem using loops and formulas.
What are Natural Numbers?
Natural numbers are the positive integers starting from 1 and going up indefinitely. In other words, they are the numbers you use for counting, such as:
1
,2
,3
,4
,5
,6
, and so on.
The sum of natural numbers refers to adding up all the numbers from 1
to a given number N
. For example, the sum of the first 5 natural numbers is:
1 + 2 + 3 + 4 + 5 = 15
How to Find the Sum of N Natural Numbers in Python
There are multiple ways to calculate the sum of the first N
natural numbers in Python. We’ll look at three different approaches:
- Using a for loop.
- Using a while loop.
- Using the mathematical formula.
Method 1: Using a For Loop
The most straightforward way to calculate the sum of natural numbers is by using a for loop to iterate from 1
to N
, adding each number to a running total.
# Program to find the sum of N natural numbers using a for loop
# Step 1: Take user input
N = int(input("Enter a number: "))
# Step 2: Initialize a variable to store the sum
sum_of_numbers = 0
# Step 3: Use a for loop to calculate the sum
for i in range(1, N + 1):
sum_of_numbers += i # Add each number to the sum
# Step 4: Print the result
print(f"The sum of the first {N} natural numbers is: {sum_of_numbers}")
Explanation of the Code
- User Input:
- We take the input number
N
from the user and convert it to an integer usingint()
.
- Initializing the Sum:
- We start by initializing a variable
sum_of_numbers = 0
to store the cumulative sum of the natural numbers.
- For Loop:
- The loop
for i in range(1, N + 1)
iterates through all numbers from1
toN
. In each iteration, the current value ofi
is added tosum_of_numbers
.
- Printing the Result:
- After the loop finishes, we print the final value of
sum_of_numbers
, which contains the sum of all natural numbers from1
toN
.
Sample Output
Enter a number: 5
The sum of the first 5 natural numbers is: 15
In this example, the sum of the first 5
natural numbers (1 + 2 + 3 + 4 + 5 = 15
) is calculated and displayed.
Method 2: Using a While Loop
You can also use a while loop to calculate the sum of natural numbers. This approach involves manually incrementing a counter and adding it to the sum.
# Program to find the sum of N natural numbers using a while loop
# Step 1: Take user input
N = int(input("Enter a number: "))
# Step 2: Initialize the sum and a counter variable
sum_of_numbers = 0
i = 1
# Step 3: Use a while loop to calculate the sum
while i <= N:
sum_of_numbers += i
i += 1 # Increment the counter
# Step 4: Print the result
print(f"The sum of the first {N} natural numbers is: {sum_of_numbers}")
Explanation of the Code
- User Input:
- The input number
N
is taken from the user.
- Initializing the Sum and Counter:
- We initialize
sum_of_numbers = 0
to store the total sum and set a counteri = 1
.
- While Loop:
- The loop runs as long as
i <= N
. In each iteration, the value ofi
is added tosum_of_numbers
, andi
is incremented by 1.
- Result:
- Once the loop ends, the sum of all numbers from
1
toN
is printed.
Sample Output
Enter a number: 6
The sum of the first 6 natural numbers is: 21
Here, the sum of the first 6
natural numbers (1 + 2 + 3 + 4 + 5 + 6 = 21
) is calculated using a while loop.
Method 3: Using the Mathematical Formula
The sum of the first N
natural numbers can also be calculated using a mathematical formula. The formula is:
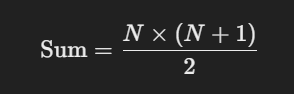
This formula provides a quick and efficient way to calculate the sum without using loops.
# Program to find the sum of N natural numbers using a mathematical formula
# Step 1: Take user input
N = int(input("Enter a number: "))
# Step 2: Use the formula to calculate the sum
sum_of_numbers = (N * (N + 1)) // 2
# Step 3: Print the result
print(f"The sum of the first {N} natural numbers is: {sum_of_numbers}")
Explanation of the Code
- User Input:
- We take the input
N
from the user.
- Formula:
- The formula
N * (N + 1) // 2
is used to calculate the sum. We use//
for integer division to ensure the result is an integer.
- Result:
- The result is printed directly after applying the formula.
Sample Output
Enter a number: 10
The sum of the first 10 natural numbers is: 55
In this case, the formula gives the sum of the first 10
natural numbers as 55
without using any loops.
Performance Comparison
While all three methods give the same result, the mathematical formula is the most efficient approach as it directly computes the sum in constant time, i.e., O(1)
. The for loop and while loop methods both have a time complexity of O(N)
, as they involve iterating through all numbers from 1
to N
.
If you’re dealing with very large values of N
, the formula method is recommended.
Conclusion
In this blog, we explored how to find the sum of the first N
natural numbers in Python using three different methods: a for loop, a while loop, and a mathematical formula. Each method helps you understand important concepts like loops and arithmetic operations, which are essential in programming.
Try out these methods yourself and see which one works best for you!
Stay tuned for more Python programming tutorials! 😊