Find the Sum of N Odd Numbers in Python
Calculating the sum of the first N
odd numbers is a great way for beginners to practice loops, conditionals, and mathematical concepts in Python. In this blog, we will explore multiple approaches to solving this problem.
By the end of this post, you’ll learn:
- What odd numbers are.
- How to calculate the sum of odd numbers using Python.
- Different methods to solve this problem using loops and formulas.
What are Odd Numbers?
Odd numbers are integers that cannot be divided by 2 without a remainder. These numbers look like:
1
,3
,5
,7
,9
,11
, and so on.
The sum of odd numbers refers to adding all the odd numbers from 1
up to a specified count. For example, the sum of the first 5
odd numbers is:
1 + 3 + 5 + 7 + 9 = 25
How to Find the Sum of N Odd Numbers in Python
There are a few different methods to calculate the sum of the first N
odd numbers in Python:
- Using a for loop.
- Using a while loop.
- Using a mathematical formula.
Method 1: Using a For Loop
A straightforward approach is to use a for loop to generate the first N
odd numbers and add them up.
# Program to find the sum of the first N odd numbers using a for loop
# Step 1: Take user input
N = int(input("Enter the value of N: "))
# Step 2: Initialize a variable to store the sum
sum_of_odds = 0
# Step 3: Use a for loop to generate and sum the odd numbers
for i in range(1, 2 * N, 2):
sum_of_odds += i # Add each odd number to the sum
# Step 4: Print the result
print(f"The sum of the first {N} odd numbers is: {sum_of_odds}")
Explanation of the Code
- User Input:
- We take input
N
from the user, which indicates how many odd numbers to sum.
- Initializing the Sum:
- The variable
sum_of_odds = 0
is used to store the cumulative sum of odd numbers.
- For Loop:
- The loop
for i in range(1, 2 * N, 2)
generates odd numbers starting from1
. Therange()
function with a step value of2
ensures we only get odd numbers. - Each odd number is added to
sum_of_odds
.
- Printing the Result:
- After the loop completes, we print the total sum of the odd numbers.
Sample Output
Enter the value of N: 5
The sum of the first 5 odd numbers is: 25
In this example, the sum of the first 5
odd numbers (1 + 3 + 5 + 7 + 9 = 25
) is calculated and displayed.
Method 2: Using a While Loop
Another way to solve this problem is by using a while loop to manually generate odd numbers and sum them.
# Program to find the sum of the first N odd numbers using a while loop
# Step 1: Take user input
N = int(input("Enter the value of N: "))
# Step 2: Initialize the sum and counter variables
sum_of_odds = 0
count = 0
current_number = 1
# Step 3: Use a while loop to sum the odd numbers
while count < N:
sum_of_odds += current_number
current_number += 2 # Move to the next odd number
count += 1 # Increment the counter
# Step 4: Print the result
print(f"The sum of the first {N} odd numbers is: {sum_of_odds}")
Explanation of the Code
- User Input:
- We take the input
N
from the user to specify how many odd numbers to sum.
- Initializing Variables:
sum_of_odds = 0
stores the sum of odd numbers.count = 0
keeps track of how many odd numbers have been added.current_number = 1
starts at the first odd number.
- While Loop:
- The loop continues as long as
count
is less thanN
. In each iteration, the current odd number is added to the sum, and bothcount
andcurrent_number
are updated.
- Result:
- After the loop ends, we print the sum of the first
N
odd numbers.
Sample Output
Enter the value of N: 4
The sum of the first 4 odd numbers is: 16
In this case, the sum of the first 4
odd numbers (1 + 3 + 5 + 7 = 16
) is calculated using a while loop.
Method 3: Using the Mathematical Formula
There is also a mathematical formula to find the sum of the first N
odd numbers, which is:
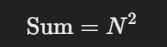
This formula provides a quick and efficient way to calculate the sum without using loops. The square of N
gives the sum of the first N
odd numbers.
# Program to find the sum of the first N odd numbers using a formula
# Step 1: Take user input
N = int(input("Enter the value of N: "))
# Step 2: Use the formula to calculate the sum
sum_of_odds = N * N
# Step 3: Print the result
print(f"The sum of the first {N} odd numbers is: {sum_of_odds}")
Explanation of the Code
- User Input:
- We take the input
N
from the user to determine how many odd numbers to sum.
- Formula:
- The sum of the first
N
odd numbers is calculated using the formulaN * N
.
- Result:
- The sum is printed directly after applying the formula.
Sample Output
Enter the value of N: 6
The sum of the first 6 odd numbers is: 36
In this example, the sum of the first 6
odd numbers (1 + 3 + 5 + 7 + 9 + 11 = 36
) is calculated using the formula.
Performance Comparison
All three methods give the same result, but the mathematical formula is the most efficient approach, with a time complexity of O(1)
(constant time). The for loop and while loop methods both have a time complexity of O(N)
because they iterate through the odd numbers to calculate the sum.
If performance is a concern, especially for large values of N
, the formula-based method is the best choice.
Conclusion
In this blog, we explored how to find the sum of the first N
odd numbers using Python. We covered three different methods: a for loop, a while loop, and a mathematical formula. Each approach teaches important concepts like loops, conditionals, and arithmetic operations, which are essential skills for Python programming.
Try these methods out and see which one works best for you!
Stay tuned for more Python programming tutorials! 😊