One of the most fundamental concepts in finance and banking is Simple Interest. It is used to calculate the interest on a loan or investment for a specified period at a fixed interest rate. In this blog post, we’ll walk through how to write a Python program to calculate simple interest.
What is Simple Interest?
Simple Interest is calculated using the formula:
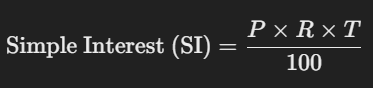
Where:
- P = Principal (the initial amount of money)
- R = Rate of Interest (annual interest rate in percentage)
- T = Time (the time period for which the interest is calculated, in years)
Simple Interest is calculated by multiplying the principal amount by the interest rate and the time period, and then dividing the result by 100.
Steps to Write the Program
- Input the Principal, Rate of Interest, and Time: We will prompt the user to input these values.
- Apply the Simple Interest Formula: Using the formula mentioned above, we will calculate the simple interest.
- Display the Result: Finally, we will print the calculated interest.
Python Program to Calculate Simple Interest
# Python program to calculate simple interest
# Input: Asking the user to enter the principal, rate of interest, and time
P = float(input("Enter the principal amount: "))
R = float(input("Enter the rate of interest: "))
T = float(input("Enter the time period in years: "))
# Calculate Simple Interest using the formula SI = (P * R * T) / 100
SI = (P * R * T) / 100
# Output: Display the simple interest
print(f"The Simple Interest is: {SI}")
Explanation:
- Input:
P
is the principal amount, input as a floating-point number to handle decimal values.R
is the annual interest rate in percentage, input as a float.T
is the time period in years for which the interest is calculated, input as a float to accommodate fractional years.
- Calculation:
- We apply the formula for Simple Interest:
SI = (P * R * T) / 100
.
- Output:
- The result,
SI
, is printed to the user.
Sample Output
Test Case 1: Simple Interest Calculation
Enter the principal amount: 5000
Enter the rate of interest: 5
Enter the time period in years: 2
The Simple Interest is: 500.0
Test Case 2: Another Example
Enter the principal amount: 10000
Enter the rate of interest: 4.5
Enter the time period in years: 3
The Simple Interest is: 1350.0
Understanding the Formula
- Principal (P): This is the initial amount of money that is either invested or loaned.
- Rate of Interest (R): This is the annual interest rate. For example, if the interest rate is 5%, then you will be charged 5% of the principal amount every year.
- Time (T): This is the duration for which the principal amount is loaned or invested.
The formula (P * R * T) / 100
gives the interest in monetary terms based on the input values. The principal remains constant, and interest is calculated at a flat rate for the given period.
Handling Edge Cases
- Negative or Zero Values:
- If any input (Principal, Rate, or Time) is zero or negative, the program will still calculate the simple interest as per the formula. However, it might not make sense in real-world applications. Example:
Enter the principal amount: 0
Enter the rate of interest: 5
Enter the time period in years: 2
The Simple Interest is: 0.0
- Decimal Values:
- The program can handle decimal values for both interest rate and time. This is useful when the time period is not a whole number or when the interest rate has decimal precision.
Modifying the Program with Exception Handling
To ensure that the program only accepts valid inputs, we can add exception handling for invalid data types:
# Python program to calculate simple interest with error handling
try:
# Input: Asking the user to enter the principal, rate of interest, and time
P = float(input("Enter the principal amount: "))
R = float(input("Enter the rate of interest: "))
T = float(input("Enter the time period in years: "))
# Calculate Simple Interest using the formula SI = (P * R * T) / 100
SI = (P * R * T) / 100
# Output: Display the simple interest
print(f"The Simple Interest is: {SI}")
except ValueError:
print("Invalid input! Please enter numeric values for principal, rate, and time.")
Now, if the user enters non-numeric values, the program will catch the ValueError
and prompt the user with a friendly error message.
Conclusion
Calculating simple interest is one of the basic and fundamental concepts in both finance and programming. With the help of a simple formula, you can easily compute how much interest you’ll earn or owe over a given period. Python makes it easy to implement this by using basic input/output and mathematical operations.
This Python program is not only a great beginner exercise but also a practical tool that can be applied to real-world financial calculations.
With this program, you’ve learned how to:
- Accept user input.
- Apply a mathematical formula in Python.
- Handle both integer and floating-point values.
Keep practicing and try enhancing this program with additional features like input validation and handling negative values!
By mastering simple programs like this, you’re building a strong foundation in Python programming that will prepare you for more complex financial and mathematical computations in the future. Happy coding!