Temperature conversion is a basic and widely-used concept in programming, especially when dealing with different unit systems. In this blog, we will cover how to convert a temperature given in Celsius to Fahrenheit using Python.
Understanding the Conversion Formula
The formula to convert Celsius to Fahrenheit is:
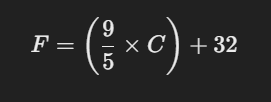
Where:
- F is the temperature in Fahrenheit.
- C is the temperature in Celsius.
This formula is derived based on the relationship between the two temperature scales, where water freezes at 0°C and 32°F, and boils at 100°C and 212°F.
Steps to Write the Program
- Input the Temperature in Celsius: We’ll prompt the user to enter a temperature in Celsius.
- Apply the Conversion Formula: Using the formula above, we’ll calculate the equivalent temperature in Fahrenheit.
- Display the Result: Finally, we will print the converted temperature.
Python Program to Convert Celsius to Fahrenheit
# Python program to convert Celsius to Fahrenheit
# Input: Asking the user to enter the temperature in Celsius
celsius = float(input("Enter the temperature in Celsius: "))
# Calculate Fahrenheit using the formula F = (9/5 * C) + 32
fahrenheit = (celsius * 9/5) + 32
# Output: Display the converted temperature
print(f"The temperature in Fahrenheit is: {fahrenheit}")
Explanation:
- Input:
celsius
: We ask the user to input a temperature in Celsius usinginput()
. We convert the input to afloat
so that it can handle decimal values.
- Calculation:
- We apply the formula to convert Celsius to Fahrenheit. The formula multiplies the Celsius value by 9/5 and then adds 32 to get the Fahrenheit equivalent.
- Output:
- The result is printed in Fahrenheit using the
print()
function.
Sample Output
Test Case 1: Positive Temperature
Enter the temperature in Celsius: 25
The temperature in Fahrenheit is: 77.0
Test Case 2: Zero Degrees
Enter the temperature in Celsius: 0
The temperature in Fahrenheit is: 32.0
Test Case 3: Negative Temperature
Enter the temperature in Celsius: -10
The temperature in Fahrenheit is: 14.0
Understanding the Conversion
- Celsius (°C): A temperature scale where water freezes at 0°C and boils at 100°C under normal atmospheric conditions.
- Fahrenheit (°F): A temperature scale primarily used in the United States, where water freezes at 32°F and boils at 212°F under normal atmospheric conditions.
The formula F = (C × 9/5) + 32
helps to translate Celsius into Fahrenheit, ensuring an accurate conversion between these two scales.
Handling Edge Cases
- Handling Extreme Temperatures:
- The program can handle any valid numeric input for Celsius, including very high and low temperatures. Example:
Enter the temperature in Celsius: 100
The temperature in Fahrenheit is: 212.0
- Invalid Inputs:
- To make the program more robust, we can add exception handling to catch invalid inputs (such as non-numeric values). Here’s how you can add error handling:
# Python program to convert Celsius to Fahrenheit with error handling
try:
# Input: Asking the user to enter the temperature in Celsius
celsius = float(input("Enter the temperature in Celsius: "))
# Calculate Fahrenheit using the formula F = (9/5 * C) + 32
fahrenheit = (celsius * 9/5) + 32
# Output: Display the converted temperature
print(f"The temperature in Fahrenheit is: {fahrenheit}")
except ValueError:
print("Invalid input! Please enter a numeric value for temperature.")
Now, if the user enters anything other than a number, the program will catch the error and display a user-friendly message.
Why is Temperature Conversion Important?
Understanding temperature conversion is essential in various fields:
- Science and Engineering: Different countries use different temperature scales, so conversions are necessary in experiments, studies, and design projects.
- Travel: When traveling to countries that use different units, it’s helpful to convert temperatures.
- Weather Applications: Weather apps often provide options to view temperatures in both Celsius and Fahrenheit, and they rely on similar conversion formulas.
Conclusion
In this blog post, we explored how to write a Python program that converts a temperature from Celsius to Fahrenheit using a simple mathematical formula. This program is not only a great exercise for beginners but also a practical tool for various real-world applications, such as weather forecasting and scientific calculations.
By understanding the relationship between Celsius and Fahrenheit, and implementing it through Python, you’ve learned how to:
- Accept user input.
- Apply a mathematical formula.
- Handle potential input errors.
Feel free to extend this program by adding features such as converting Fahrenheit to Celsius, or implementing other unit conversions. Keep practicing, and happy coding!